In Scala, a tuple is used to store fixed and different types of distinct data. It is immutable and used when there is a need to return multiple values from methods.
The following is an example of a Tuple that is holding the string and integer both data.
val t = ("hello", 30)
The data type of tuple is dependent upon the elements it contains. In the above example tuple elements are string and integer both so tuple data type would be tuple [string, int].
How to access Tuples
We can access elements of a Tuple t using method t._1 it will access the first element, t._2 it will access the second element, and so on.
Let us see with the below example. We have created class flowers and assigned values to tuples after that accessing the elements of tuples using t._1, t._2, t._3.
case class Flowers (name: String)
val t = (1, "One", Flowers ("Rose"))
val t = (2, "Two", Flowers ("Orchid"))
val t = (3, "Three", Flowers ("Jasmine"))
Output
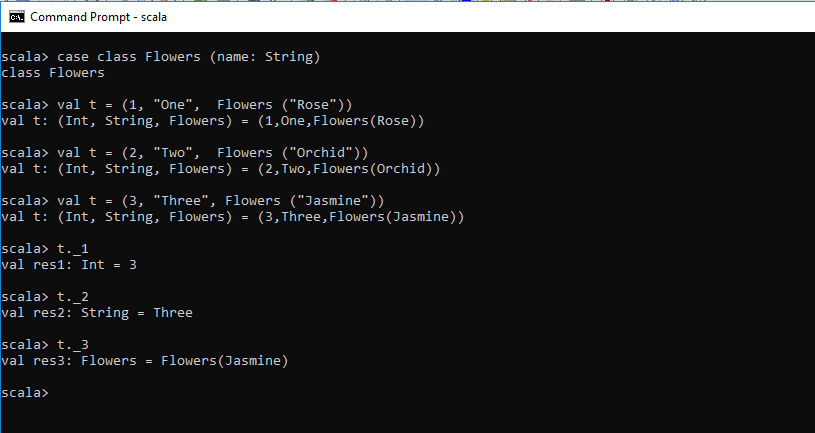
Let us see another example by writing a method that takes a list and returns the sum. Save the program with tupleexample.scala name and run it from the terminal by using scala tupleexample.scala command(refer screenshot).
object tupleexample {
def main(args: Array[String]) {
val tup = (1,5,5,4)
val sum = tup._1 + tup._2 + tup._3 + tup._4
println( "Result:" + sum )
}
}
Output
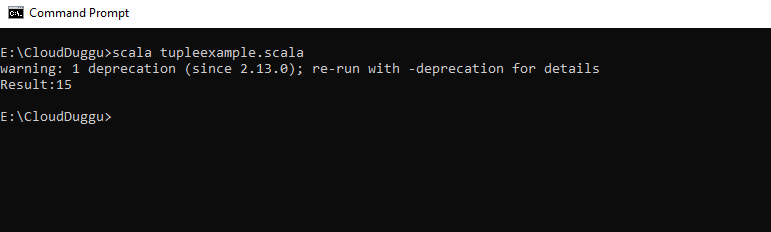
Click Here To Download Code File.
Tuple Swap Method
By using the swap method we can swap the element of tupel2.
Let us see with the below example to swap elements. Save the program with tupleswap.scala name and run it from the terminal by using scala tupleswap.scala command(refer screenshot).
object tupleswap {
def main(args: Array[String]) {
val t = new Tuple2("World", "hello")
println("Swapped Tuple: " + t.swap )
}
}
Output
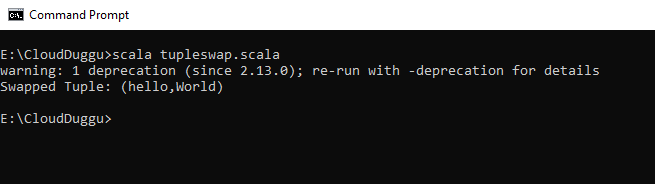
Click Here To Download Code File.
Tuple productIterator Method
By using the Tuple.productIterator() method we can iterate over all the elements of a Tuple.
Let us see with the below example to iterate elements. Save the program with tupleiterate.scala name and run it from the terminal by using scala tupleiterate.scala command(refer screenshot).
object tupleiterate {
def main(args: Array[String]) {
val t = (1,2,3,4,5,6,7,8,9,10)
t.productIterator.foreach{ i =>println("Result = " + i )}
}
}
Output
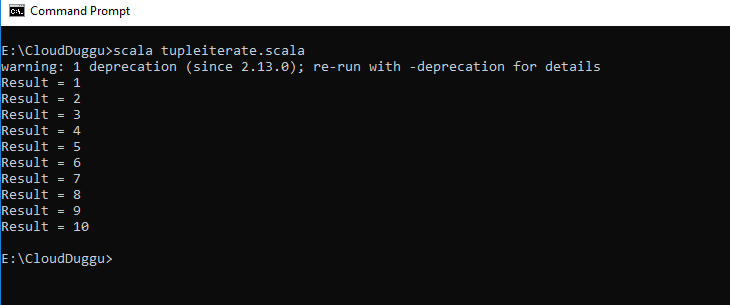
Click Here To Download Code File.