What are the Data types in Scala?
In Scala, every value is assigned a type such as numerical values and functions. The below diagram shows a subset of the type hierarchy.
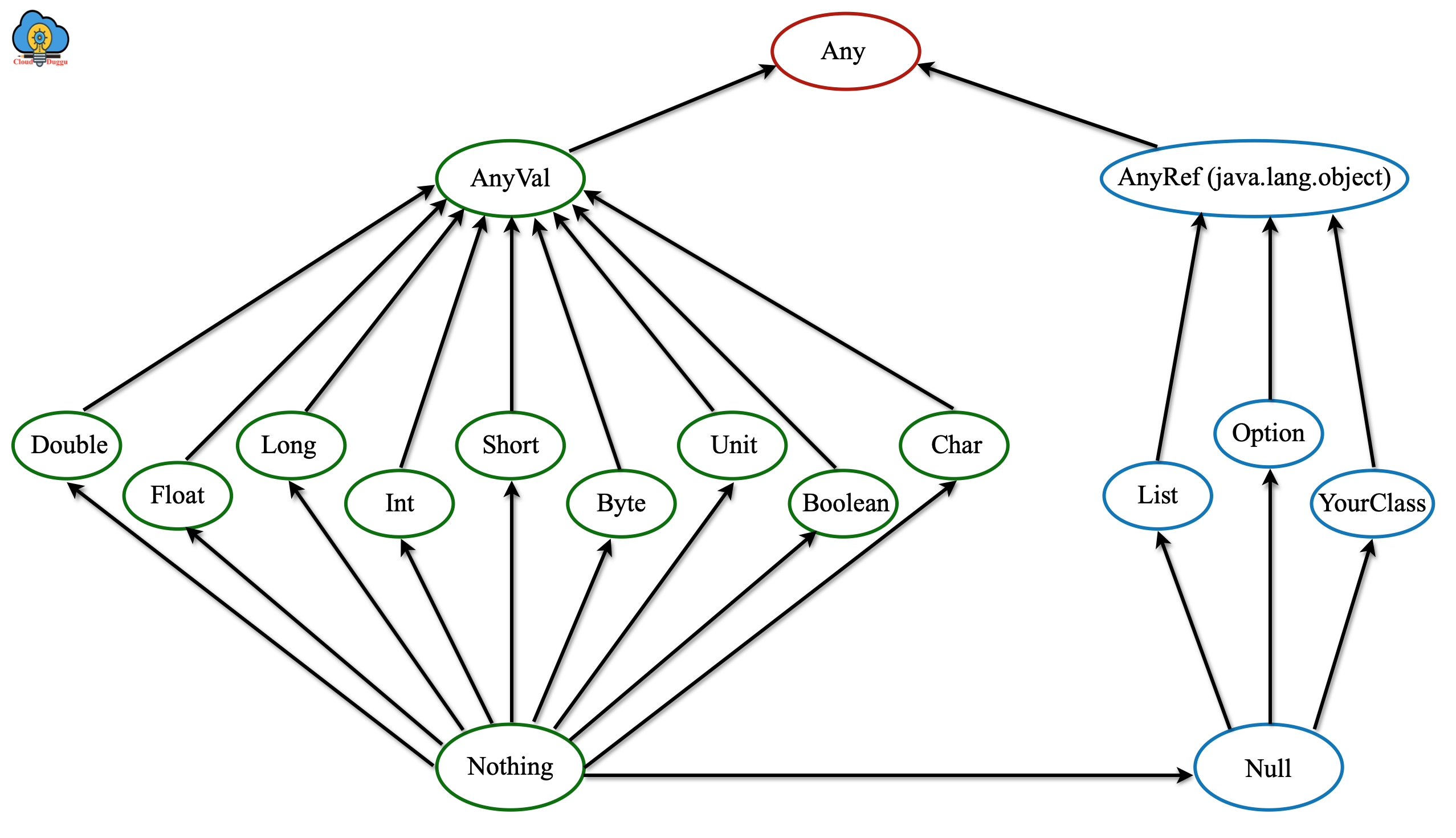
At the top Scala has any data type that is used to define some universal methods that are hashCode, equals, and toString. The top data type has direct subclasses such as AnyVal and AnyRef. AnyVal is used to represents the value types. Apart from this, it has nine predefined value types which are on-nullable such as Float, Double, Char, Long, Byte, Int, Boolean, and Short. The user type defined in Scala is a subtype of AnyRef.
Let us understand it with the below example.
In the following program, the characters, integers, functions, and boolean values are the objects and a value list of type List[Any] is defined. Now the list is initialized from different types of elements and accepted by the list.
val list: List[Any] = List(
"a string",
732, // an integer
'c', // a character
true, // a boolean value
() => "an anonymous function returning a string"
)
list.foreach(element => println(element))
Output of the program.
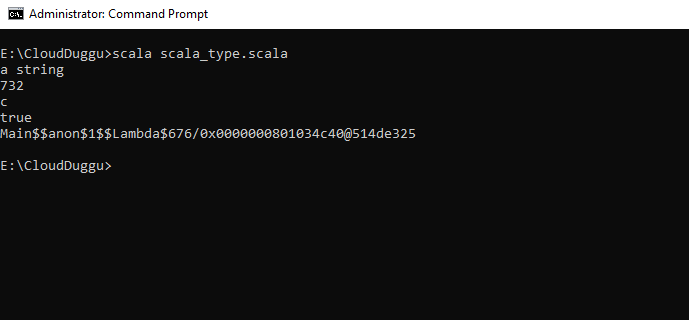
Click Here To Download Code File.
Scala Data Types
Scala supports the following list of data types.
1. | Byte | signed value 8 bit . Range from -128 to 127 |
2. | Short | signed value 16 bit . Range from -32768 to 32767 |
3. | Int | signed value 32 bit . Range from -2147483648 to 2147483647 |
4. | Long | signed value 64 bit . from -9223372036854775808 to 9223372036854775807 |
5. | Float | IEEE 754 single-precision float 32 bit |
6. | Short | IEEE 754 double-precision float 64 bit |
7. | Char | unsigned Unicode character 16 bit . Range from U+0000 to U+FFFF |
8. | String | A sequence of Chars |
9. | Boolean | Either the literal true or the literal false |
10. | Unit | Corresponds to no value |
11. | Null | null or empty reference |
12. | Nothing | The subtype of every other type; includes no values |
13. | Any | The supertype of any type; any object is of type Any |
14. | AnyRef | The supertype of any reference type |