In Scala, Methods usually accepts multiple parameter lists but in case a method is called with less parameter then it throws a missing parameter list that is called currying. By using Curring a function can take multiple arguments in a sequence of functions and each function tasks a single argument.
Scala Multiple parameter groups provide the following benefits.
- It allows implicit and non-implicit both types of parameters.
- They facilitate type inference.
- The parameter can be used in multiple groups.
Let us see the foldLeft method example that applies a two-parameter function op to an initial value z and all elements of this collection, going left to right.
val numbers_data = List(3,6,5,3,6,1,2,8,7,5,9,10)
val result_output = numbers_data.foldLeft(0)((p, q) => p + q)
println(result_output)
Output

Use Cases for Multiple Parameter Lists
The following are see some of the use cases of multiple parameter lists.
Drive Type Inference
In Scala, type inference precedes one parameter list at a time. If you have the following method.
def foldLeft1_def[S, T](as: List[S], b0: T, op: (S, T) => T) = ???
And you are trying to call it this way then it doesn’t compile.
def notPossible_def = foldLeft1_def(numbers, 0, _ + _)
You can call it as mentioned below because Scala won’t be able to infer the type of the function _ + _, as it’s still inferring Y and Z.
def foldLeft2_def[E, F](as: List[E], b0: F)(op: (F, E) => F) = ???
def possible = foldLeft2_def(numbers, 0)(_ + _)
The above definition will not require any hints and understand all its parameters.
Implicit Parameters
In Scala, to specify only certain parameters as implicit, they must be placed in their implicit parameter list.
def execute(arg: Int)(implicit ec: scala.concurrent.ExecutionContext) = ???
Partial Application
A Partial Application comes in a condition If a method is called with less number of arguments then it will produce a function that will take the missing parameters as arguments.
Let us see with the below example.
val numbers_data = List(9,8,7,8,3,6,5,2,4,1,7,10)
val numberFunc_val = numbers_data.foldLeft(List[Int]()) _
val squares_val = numberFunc_val((ex, e) => ex :+ e*e)
println(squares_val)
val cubes_val = numberFunc_val((ex, e) => ex :+ e*e*e)
println(cubes_val)
Output
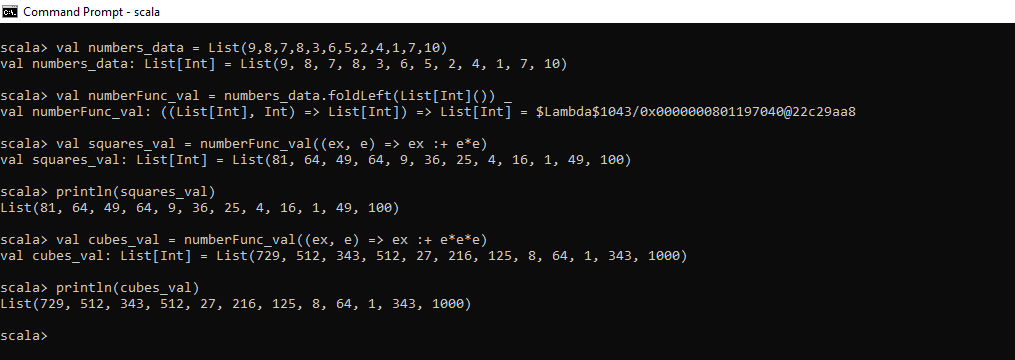