In a programming language, pattern matching is a mechanism through which a value is checked against a pattern of values. The value of the pattern is mostly a tree structure or a sequence of values. The use case of pattern matching is projecting the location of a pattern in a token of sequence.
The following example will match and display a value against patterns that have different types.
object pattermatchexp_data {
def main(args: Array[String]) {
println(matchresult_out(10))
}
def matchresult_out(d: Int): String = d match {
case 0 => "zero"
case 1 => "one"
case 2 => "two"
case _ => "other"
}
}
Output
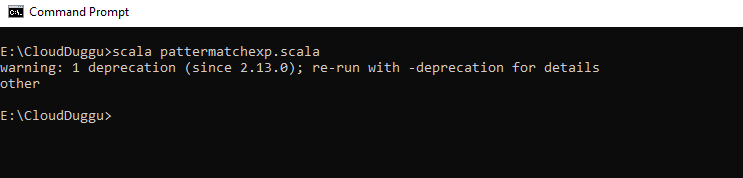
Click Here To Download Code File.
The following example will match and display a value against patterns that have different types.
object pattermatchexmp2_data{
def main(args: Array[String]) {
println(matchresult_out("two"))
println(matchresult_out("test"))
println(matchresult_out(1))
}
def matchresult_out(u: Any): Any = u match {
case 1 => "one"
case "two" => 2
case x: Int => "scala.Int"
case _ => "many"
}
}
Output
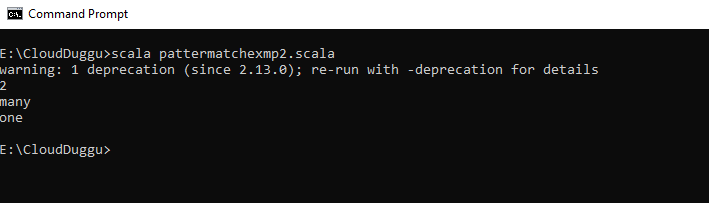
Click Here To Download Code File.
Pattern Matching with Case Classes
Case Classes are especially useful for pattern matching. When we add Case Class in the program then it will automatically add methods like equals, hashCode, and toString to the class and after that, we don’t need to add toString() methods.
The following is an example of pattern matching with Case Classes.
object patterncaseexmple {
def main(args: Array[String]) {
val krish = new personname_data("krish", 30)
val joan = new personname_data("joan", 40)
val wick = new personname_data("wick", 39)
val herry = new personname_data("herry", 25)
for (personname_data <- List(krish, joan, wick, herry)) {
personname match {
case personname_data("krish", 30) => println("Hi Krish! How are you?")
case personname_data("joan", 40) => println("Hi Joan! How are you?")
case personname_data("wick", 39) => println("Hi Wick! How are you?")
case personname_data(username, user_age) =>
println("User_Age: " + user_age + "Age_in_year, user_name: " + username + "?")
}
}
}
case class personname_data(username: String, user_age: Int)
}
Output
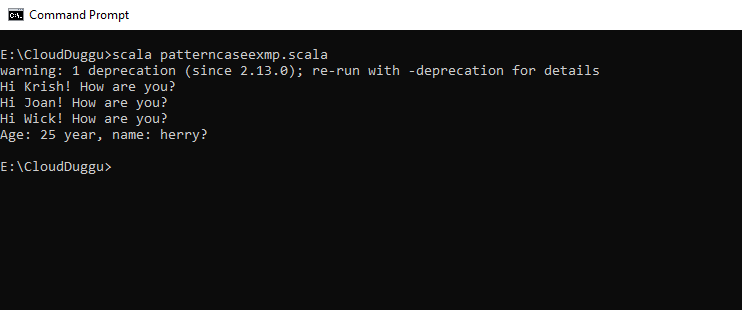
Click Here To Download Code File.