In Scala, Operators are the methods that are used to perform some actions like Arithmetic Operators that perform Arithmetic operations, Logical Operators that are used to perform logical operations, and so on.
Let us see each operator in detail.
1. Arithmetic Operators
Arithmetic Operators are used to perform arithmetic operations. We have below a list of Arithmetic Operators supported by Scala.
Operations | Actions |
---|---|
+ | This operator adds two operands. |
- | This operator subtracts the second operand from the first. |
* | This operator multiplies both operands. |
/ | This operator divides numerator by de-numerator. |
% | This operator returns the remainder. |
Arithmetic Operators Examples
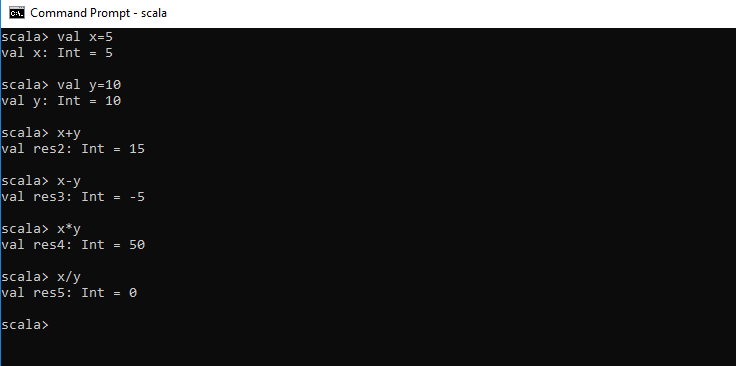
2. Relational Operators
Relational Operators have been used to defines some kind of relation between two entities. We have below a list of Relational Operators supported by Scala.
Operations | Actions |
---|---|
== | This operator returns true if the two values are equal otherwise it will return false. |
! = | This operator returns true if the two values are unequal otherwise it will return false. |
> | This operator returns true if the first operand is greater than the second; otherwise it will return false. |
< | This operator returns true if the first operand is lesser than the second; otherwise it will return false. |
> = | This operator returns true if the first operand is greater than or equal to the second otherwise it will return false. |
<= | This operator returns true if the first operand is less than or equal to the second otherwise it will return false. |
Relational Operators Examples
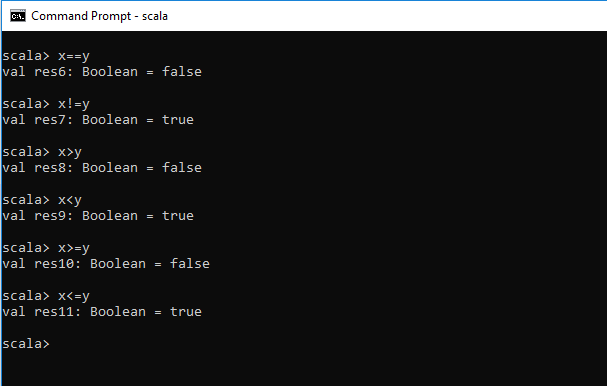
3. Logical Operators
Logical Operators are used to performing logical operations. We have below a list of Logical Operators supported by Scala.
Operations | Actions |
---|---|
&& | This operator returns true if both operands are true otherwise it will return false. |
|| | This operator returns true if either or both operands are true otherwise it will return false. |
! | This operator reverses the operand’s logical state, if it is true then false and if it is false then true. |
Logical Operators Examples
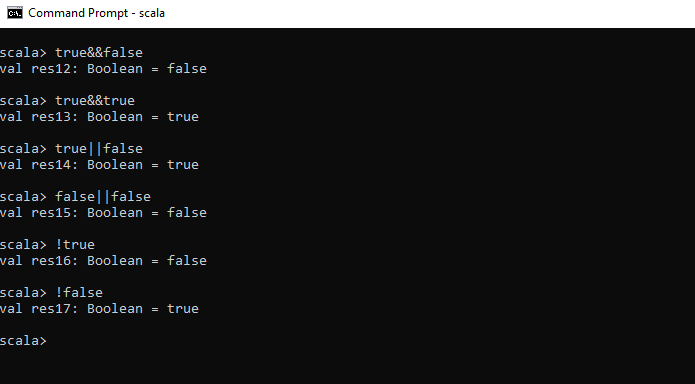
4. Bitwise Operators
In Scala we have 7 Bitwise operators, let us see the below table and then we see the Bitwise operators example.
p | q | p&q | p|q | p^q |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
Operations | Actions |
---|---|
& | This operator performs an AND operation on each pair of bits in the binary equivalents for the two operands. |
| | This operator performs an OR operation on each pair of bits in the binary equivalents for the two operands. |
^ | This operator performs an XOR operation on each pair of bits in the binary equivalents for the two operands. |
~ | This operator flips the bits in the operand’s binary equivalent. |
<< | This operator moves the bits of the left operand by (the right operand) number of bits to the left. |
>> | This operator moves the bits of the left operand by (the right operand) number of bits to the right. |
>>> | This operator moves the bits of the left operand by (the right operand) number of bits to the right and fills these places with zeroes |
Bitwise Operators Examples
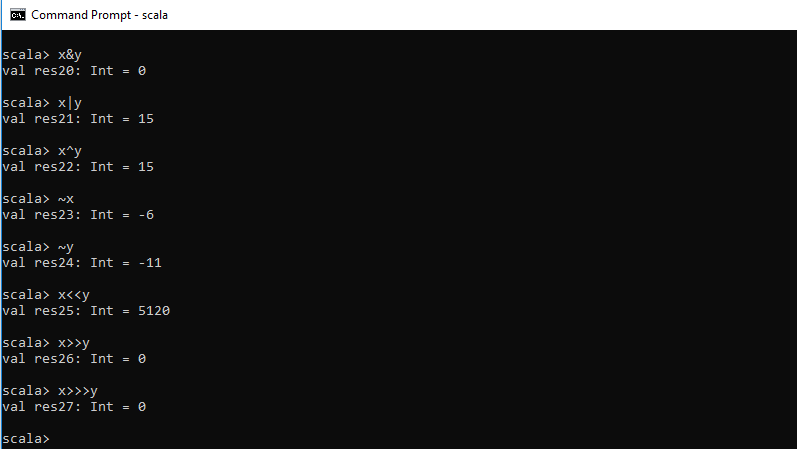
5. Assignment Operators
Assignment Operators are used to performing Assignment operations. We have below a list of Assignment Operators supported by Scala.
Operations | Actions |
---|---|
= | This operator assigns the value on the right to the operand on the left. |
+ = | This operator performs the addition and assigns value. |
- = | This operator subtracts the second operand from the first, then assigns the result to the left operand. |
* = | This operator multiplies the two operands and then assigns the result to the left operand. |
/ = | This operator divides the first operand by the second and then assigns the result to the left operand. |
% = | This operator calculates the modulus remaining after dividing the first operand by the second and assign value. |
<< = | This operator shifts the left operand by some bits to the left. Then, it assigns the result to the left operand. |
>> = | This operator shifts the left operand by some bits to the right. Then, it assigns the result to the left operand. |
& = | This operator applies the bitwise AND operator on the two operands and then assigns the result to the left operand. |
^ = | This operator performs bitwise XOR operation on the two operands, and then assigns this result to the left operand. |
| = | This operator performs a bitwise-inclusive-OR operation on the two operands, and then assigns this result to the left operand |
Scala Operator Precedence
Below is the list of Scala Operator Precedence which is used when an expression uses multiple operators and then operators are evaluated based on the priority of the first character.
Operators | Operator Type | Operator Precedence |
---|---|---|
() [] | Postfix Operators | Left to Right |
! ~ | Unary Operators | Right to Left |
* / % | Multiplicative Operators | Left to Right |
+ – | Additive Operators | Left to Right |
>> >>> << | Shift Operators | Left to Right |
> >= < <= | Relational Operators | Left to Right |
== != | Equality Operators | Left to Right |
& | Bitwise AND Operator | Left to Right |
^ | Bitwise XOR Operator | Left to Right |
| | Bitwise OR Operator | Left to Right |
&& | Logical AND Operator | Left to Right |
|| | Logical OR Operator | Left to Right |
= += -= *= /= %= >>= <<= &= ^= |= | Assignment Operators | Right to Left |
, | Comma Operator | Left to Right |