Introduction of Scala Collections
The Scala collection the placeholder where we can put items such as List, Set, Tuple, Option, Map, etc. Scala provides supports for two types of collections mutable collections and immutable collections. In a Mutable collection, elements can be easily added, change, or remove whereas in the immutable collection if we perform these operations then a new collection is returned, and the old collection remains as it is.
Features of Scala Collections
The following are some of the features of Scala Collections.
1. Fast Operation
Collection operations are optimized in its libraries and using the PAR method we can run a sequential collection into a parallel that provides fast operations.
2. Universal
In Scala, collection performs the same operation on any type. For example, a string is conceptually a sequence of characters also it supports all operations.
The following code shows many advantages of Scala’s collections.
The following is an example of the collection in which an employee collection is partitioned in Engineer and SrEngineer based on their salary so the type of Engineer and SrEngineer would be the same as of employee.
val (Engineer, SrEngineer) = people partition (salary < 5000)
3. Concise
In Scala, we can use the lightweight syntax to perform functional operations that return the result as a custom algebra.
Mutable and Immutable Collections
The collections in Scala are divided into two parts, the first one is called mutable collections and the second one is called immutable collections. We can perform the update, add, remove the mutable collection whereas Immutable collections don't change hence we perform any action on that then it creates a new collection leaving the old collection as it is.
We can find all collection classes under scala.collection package.
Let us see mutable and immutable collections in detail.
Mutable Collections
In Scala, a Mutable collection is present in scala.collection.mutable package and easily created. If we are using Mutable collect then need to understand the code properly and the changes it is doing for collections.
Immutable Collections
The Immutable collections are those which are created once and never change. It is present under scala.collection.immutable package. If we perform any action on Immutable collections then it will produce a new collection.
In Scala, there is a root collection that is present in the package of scala. collection. It is used to provide the same interface as immutable collections but the major difference between these two is that immutable collections can't be changed by any of the clients whereas the client can give a promise not to change the collection for root collections and Scala chooses immutable collections always.
The following figure shows all collections present under scala.collection package.
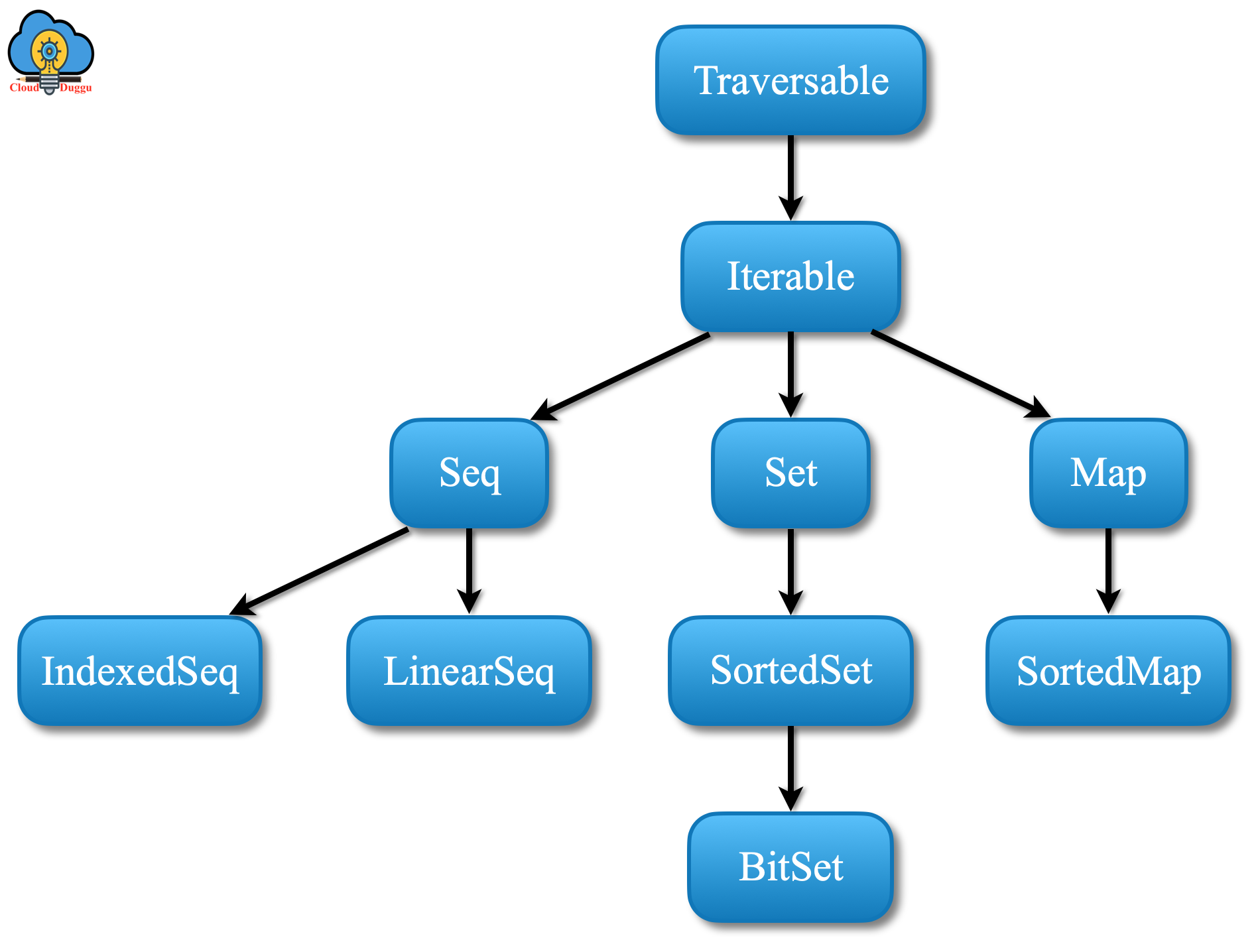
Scala Collection and Operations
The following are the commonly used Scala Collections and their types.
1. Scala - Sets Collection
Scala Set collection contains a unique value of a similar type. There are two types of Set collection which are set immutable collection and set mutable collection and Scala uses immutable set by default. The package scala.collection.mutable.Set class is used to use mutable set collections.
Scala Sets Basic Operations
Let us see some basic operations on set collections with the below example.
Additions
In addition, you can use + and ++ to add one or more elements to a set.
Let us understand with the below example.
Please save the below piece of code with name additionsets.scala and run it from the Scala terminal.
object additionsets {
def main(args: Array[String]) {
val flowerName1 = Set("Aconitum", "Agapanthus", "Alchemilla","Alstroemeria")
val flowerName2 = Set("Alstroemeria", "Alyssum")
var flowerName = flowerName1 ++ flowerName2
println( "flowerName1 ++ flowerName2:" + flowerName)
flowerName = flowerName1.++( flowerName2)
println( "flowerName1.++( flowerName2) :" + flowerName)
}
}
Output
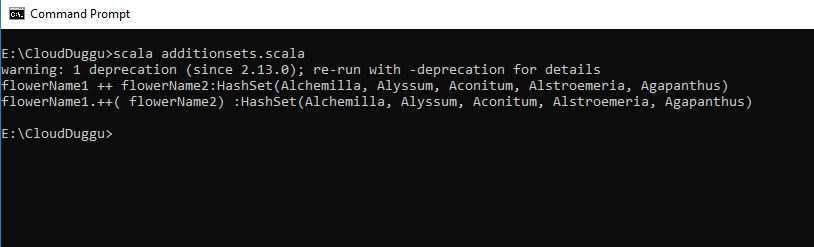
Click Here To Download Code File.
Removals
For Removals, you can use - and -- to remove one or more elements from a set.
Let us understand with the below example.
Please save the below piece of code with name removalsets.scala and run it from Scala terminal.
object removalsets {
def main(args: Array[String]) {
val flowerName1 = Set("Aconitum", "Agapanthus", "Alchemilla","Alstroemeria","Amaryllis","Gazania")
val flowerName2 = Set("Alstroemeria", "Alyssum","Gazania")
var flowerName = flowerName1 -- flowerName2
println( "flowerName1 -- flowerName2:" + flowerName)
flowerName = flowerName1.--( flowerName2)
println( "flowerName1.--( flowerName2) :" + flowerName)
}
}
Output
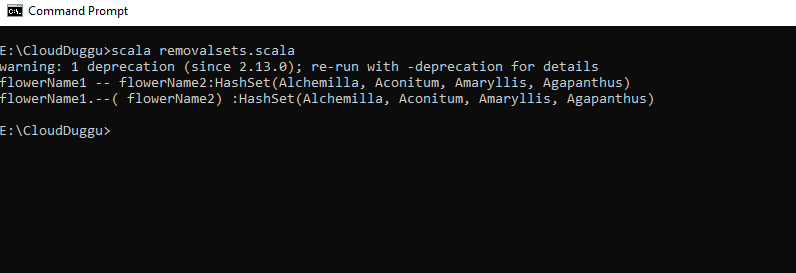
Click Here To Download Code File.
2. Scala - Map Collection
The Scala Map collection is the collection type of key and value. If we want to access values then we can use its key for that. There are two types of Map collection, mutable Map collection and immutable map collection and Scale uses immutable map collections default. The package scala.collection.mutable.msp class is used to use the mutable Map collection.
Let us see some basic operations on Map collections with the below example.
Scala map Basic Operations
Let us see some basic operations on map collections with the below example.
Additions
In addition, you can use + and ++ to add new bindings to a map or change existing bindings.
Let us understand with the below example.
Please save the below piece of code with the name scala_mapaddition.scala and run it from the Scala terminal.
object scala_mapaddition{
def main(args: Array[String]) {
val flowerName1 = Map("Aconitum" -> "Ranunculus Family", "African" -> "Gazania" , "Alchemilla" ->
"Lady's Mantle")
val flowerName2 = Map("Alstroemeria" -> "Lily", "Alyssum -> "Sweet Alyssum","African" -> "Gazania")
var flowerName = flowerName1 ++ flowerName2
println( "flowerName1 ++ flowerName2:" + flowerName)
flowerName = flowerName1.++( flowerName2)
println( "flowerName1.++( flowerName2) :" + flowerName)
}
}
Output
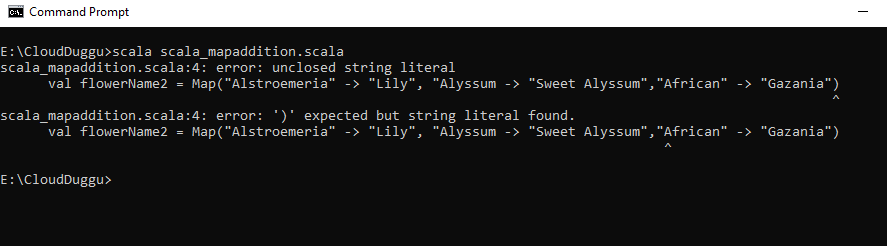
Click Here To Download Code File.
3. Scala – Array Collection
An Array collection is a special type of collection in Scala. It is a data structure that contains the collection of elements and each element is identified by an index and a key.
scala> val a1 = Array(1, 2, 3,4,5,6,7,8,9,10)
val a1: Array[Int] = Array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
scala> val a2 = a1 map (_ * 2)
val a2: Array[Int] = Array(2, 4, 6, 8, 10, 12, 14, 16, 18, 20)
scala> val a3 = a2 filter (_ % 5 != 0)
val a3: Array[Int] = Array(2, 4, 6, 8, 12, 14, 16, 18)
scala> a3.reverse
val res0: Array[Int] = Array(18, 16, 14, 12, 8, 6, 4, 2)
Output
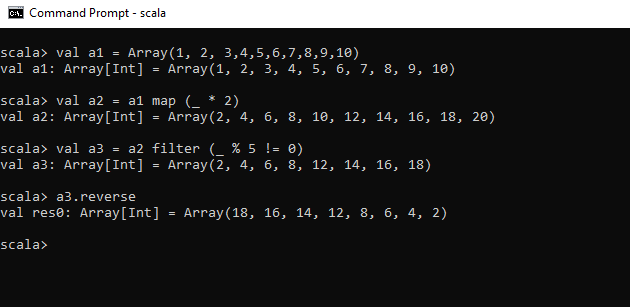