What is Case Class in Scala?
In Scala, case classes are used to shape the immutable data and use pattern matching to decompose it. It is similar to regular classes and the parameter used in Case Class is immutable and public.
When we define a Class as a Case Class, then we don’t need to use the new keyword to create a new instance.
case class Person(name: String, relation: String)
What is Case Object in Scala?
In Scala, a Case object is also an object that is defined with a “case” modifier.
val krish = Person("krish", "joshi")
Case Class Benefits
The following are some of the benefits of Scala Case Class.
- Scala Compiler adds toString, hashCode, and equals methods by default to avoid manual writing.
- Scala Compiler adds copy method by default.
- Scala Case classes are easily used in Pattern Matching.
- In Scala, the Case objects & class are by default Serializable
- We can define Algebraic data types by using Case Classes.
Case Class without the new keyword
The following examples show that we can see that Case Class is created without the “new” keyword, It is allowed to use the “new” keyword, but not recommended.
case class Person(name: String, relation: String)
val krish = Person("krish", "joshi")
Output
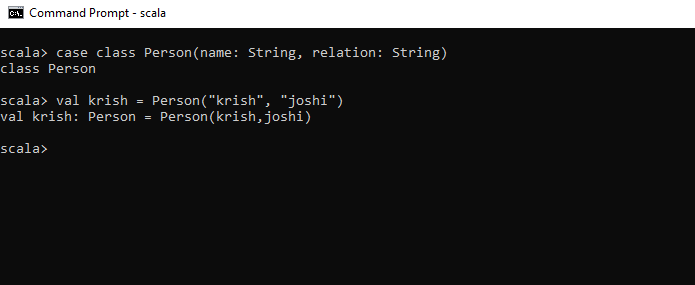
Case Class without mutator methods
In Scala, the default constructor parameters of Case Class are Val and hence for each parameter, the accessor method is created but not the mutator methods.
krish.name
val res8: String = krish
krish.name="joan"
error: reassignment to val
Output
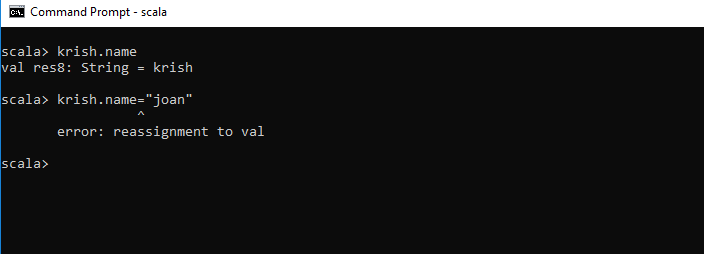
Case Class unapply methods
In Scala, The unapply method is created automatically in Vase Class.
krish match { case Person(n, r) => println(n, r) }
Output
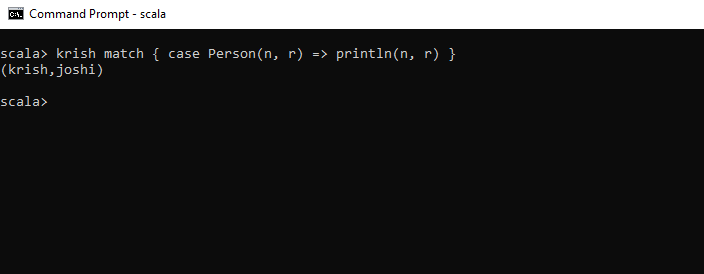
Case Class copy method
The copy method is used in cloning the object so that any change in fields can be performed.
case class WorldCupteam(name: String, lastWorldSeriesWin: Int)
val team2019 = WorldCupteam("England", 2019)
val team2015 = team2019.copy(lastWorldSeriesWin = 2015)
Output
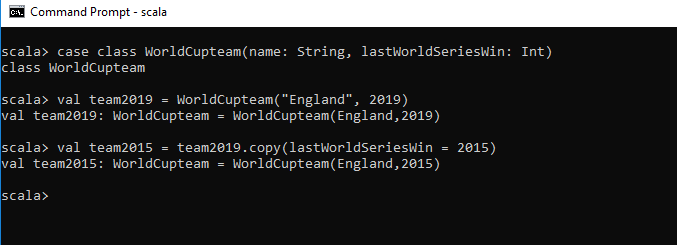
Case Class equals and hashCode methods
By using equals and hashCode methods we can easily use our objects in collections like sets and maps.
val John = Person("John", "Wick")
krish == John
Output
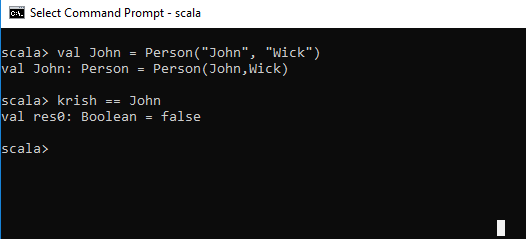
Case Class equals toString methods
toString is a default method that is used in debugging a code. If we just write down the variable name it will show it's class and variable name.
krish
val res1: Person = Person(krish,joshi)
Output
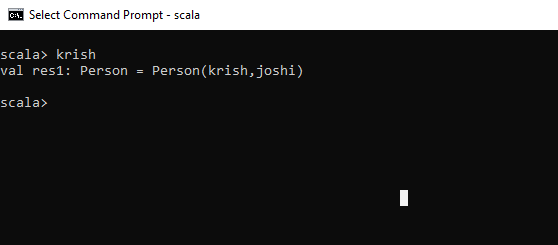
How to check Code Generated by Case Class?
We can see the code that Scala case classes generate. To do that, first, compile a simple case class, then disassemble the resulting .class files with javap.
Create a scala file with the name caseclasscode.scala and put below statement.
case class caseclasscode(var name: String, var age: Int)
Compile the code from the command prompt. Please use the below command to compile.
scalac caseclasscode.scala
scalac creates two JVM class files, caseclasscode.class and caseclasscode $.class. Disassemble caseclasscode.class with this command.
Use javap to see the public signature of the class
javap caseclasscode
Output
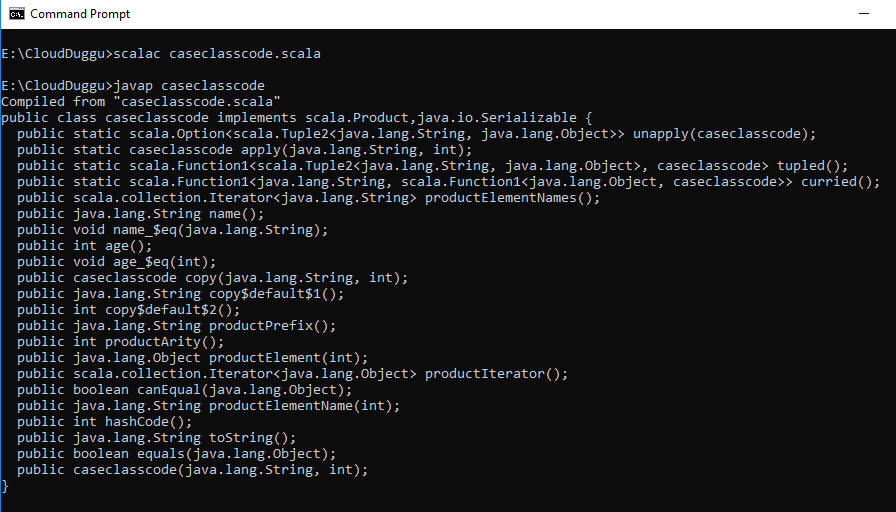
Click Here To Download Code File.