MongoDB provides the find() method to select the Documents from a Collection. The find() method is similar to the Select operation of SQL. For example, if we want to see all records from the "inventory" collection then we will write db.inventory.find( {} ) in MongoDB, and in SQL we can write SELECT * FROM inventory.
Now let us see the find() method in detail.
db.collection.find()
The find() method is used to return the result set of a collection. It uses the cursor to display the first 20 results and then if you press enter then again it will iterate and display the records.
Syntax:
The following is the syntax of find() method.
Let us see an example to create a collection with the name "inventory_records" in the "mongodb_test" database that we have already created in the database section and insert the below Documents in the collection. Once the Documents are inserted then we will use the find() method to project the Documents.
Example :
db.inventory_records.insertMany([
{
"item_name":"canvas",
"item_qty":110,
"item_size":{
"height":29,
"weight":36.5,
"uom":"cm"
},
"status":"A"
},
{
"item_name":"journal",
"item_qty":35,
"item_size":{
"height":15,
"weight":22,
"uom":"cm"
},
"status":"A"
},
{
"item_name":"mat",
"item_qty":95,
"item_size":{
"height":23.9,
"weight":32.5,
"uom":"cm"
},
"status":"A"
},
{
"item_name":"mousepad",
"item_qty":45,
"item_size":{
"height":16,
"weight":28.85,
"uom":"cm"
},
"status":"P"
},
{
"item_name":"notebook",
"item_qty":60,
"item_size":{
"height":7.5,
"weight":10,
"uom":"in"
},
"status":"P"
},
{
"item_name":"paper",
"item_qty":200,
"item_size":{
"height":9.5,
"weight":12,
"uom":"in"
},
"status":"D"
},
{
"item_name":"planner",
"item_qty":75,
"item_size":{
"height":25.85,
"weight":40,
"uom":"cm"
},
"status":"D"
},
{
"item_name":"postcard",
"item_qty":25,
"item_size":{
"height":15,
"weight":17.25,
"uom":"cm"
},
"status":"A"
},
{
"item_name":"sketchbook",
"item_qty":90,
"item_size":{
"height":13,
"weight":23,
"uom":"cm"
},
"status":"A"
},
{
"item_name":"sketch_pad",
"item_qty":85,
"item_size":{
"height":25.85,
"weight":32.5,
"uom":"cm"
},
"status":"A"
}
]);
This operation can be written as below in SQL Language.
Output:
In the below figure we can see the Documents are inserted in the "inventory_records" Collection using the insertmany() method.
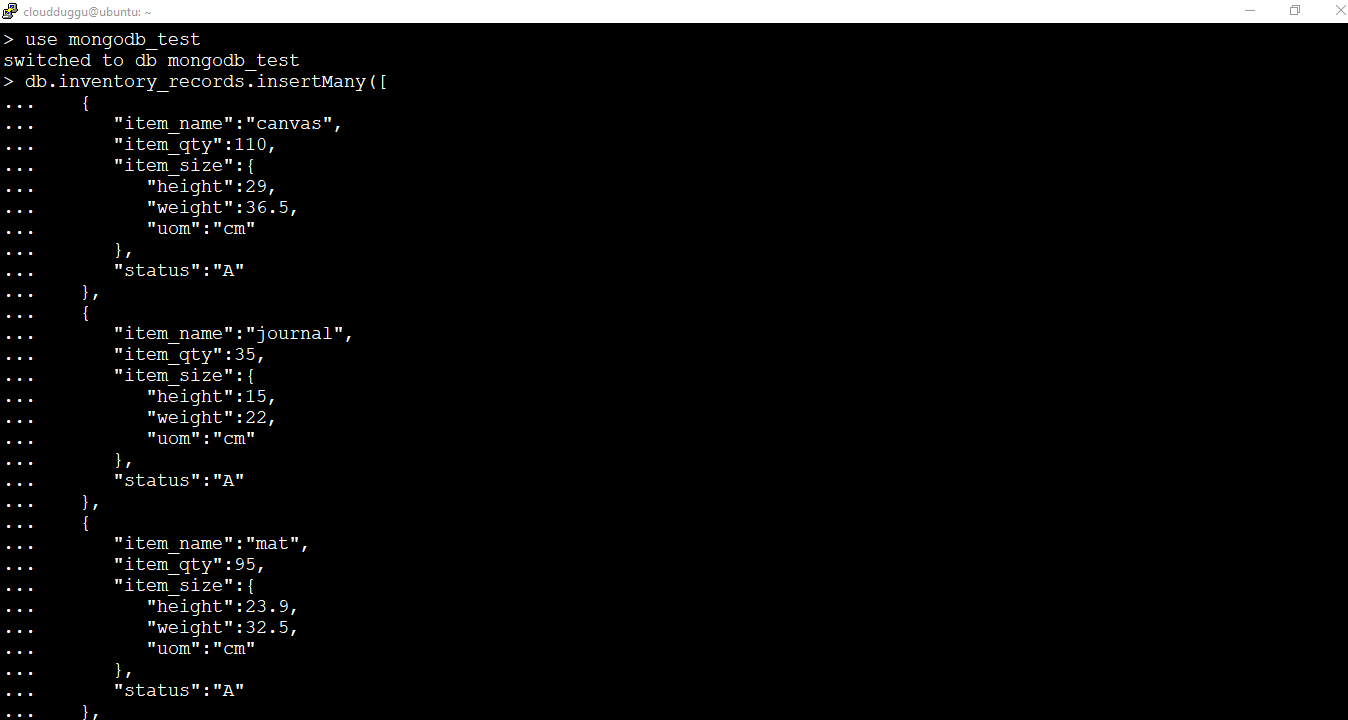
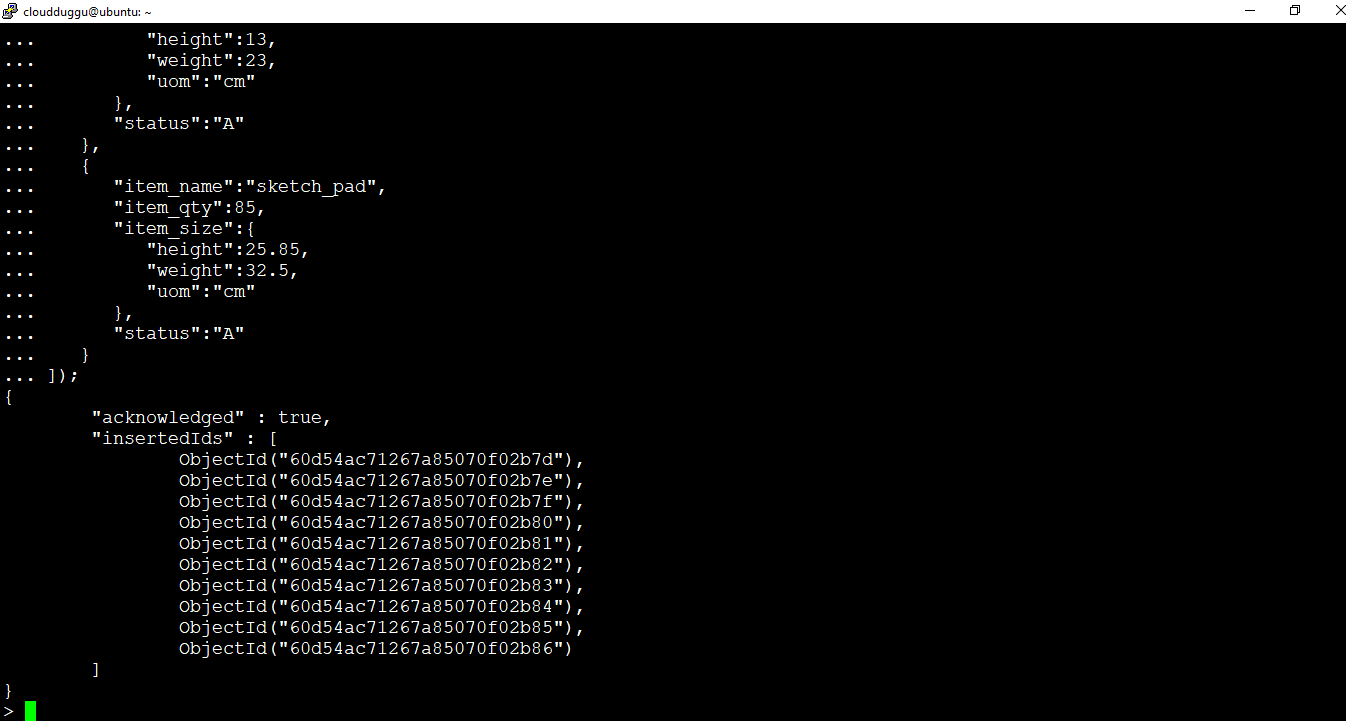
In the below figure, we can see that the find() method has projected all Documents from the "inventory_records" Collection.
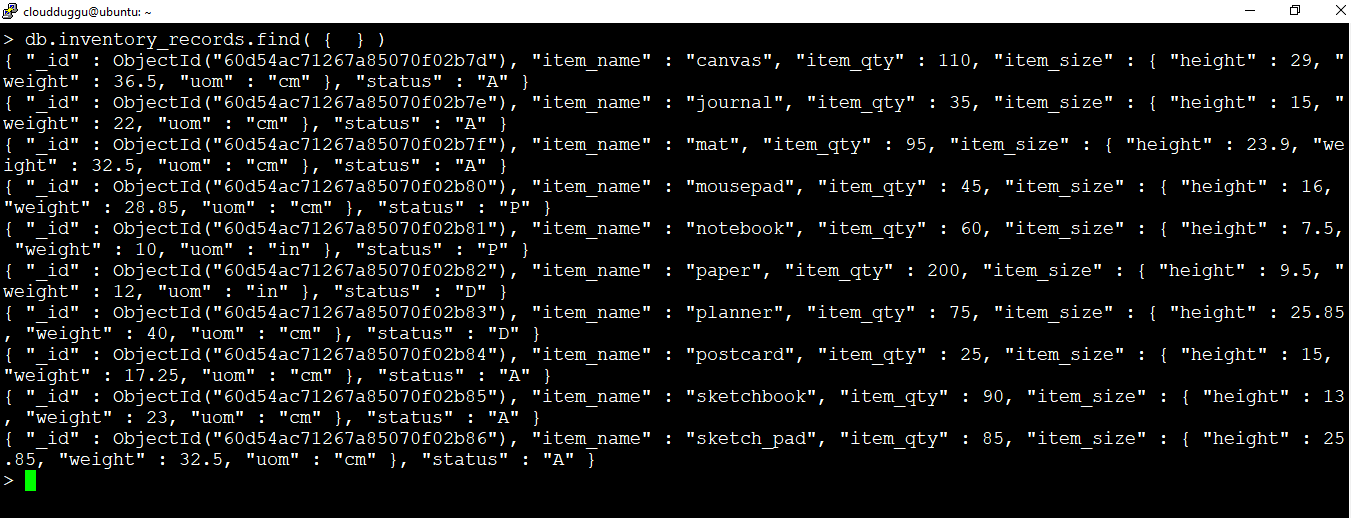
db.collection.findOne()
The findone() method is used to return only one Document.
Syntax:
The following is the syntax of pretty() method.
In the below example we will use the findone() method to find out the matching records where item_name = "canvas".
Example:
We can write the same operation in SQL language as mentioned below.
Output:
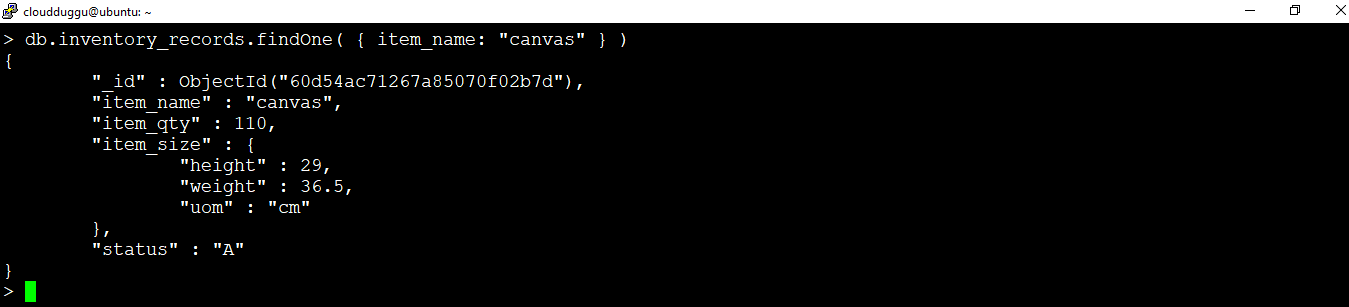
db.collection.find().pretty()
The pretty() method is used to show the result in an easy-to-read format.
Syntax:
The following is the syntax of pretty() method.
Let us see the below example to show the "inventory_records" Collection Documents in a readable format using the pretty() method.
Example:
Output:
We can see from the below output that the Documents are returned in a formatted way.
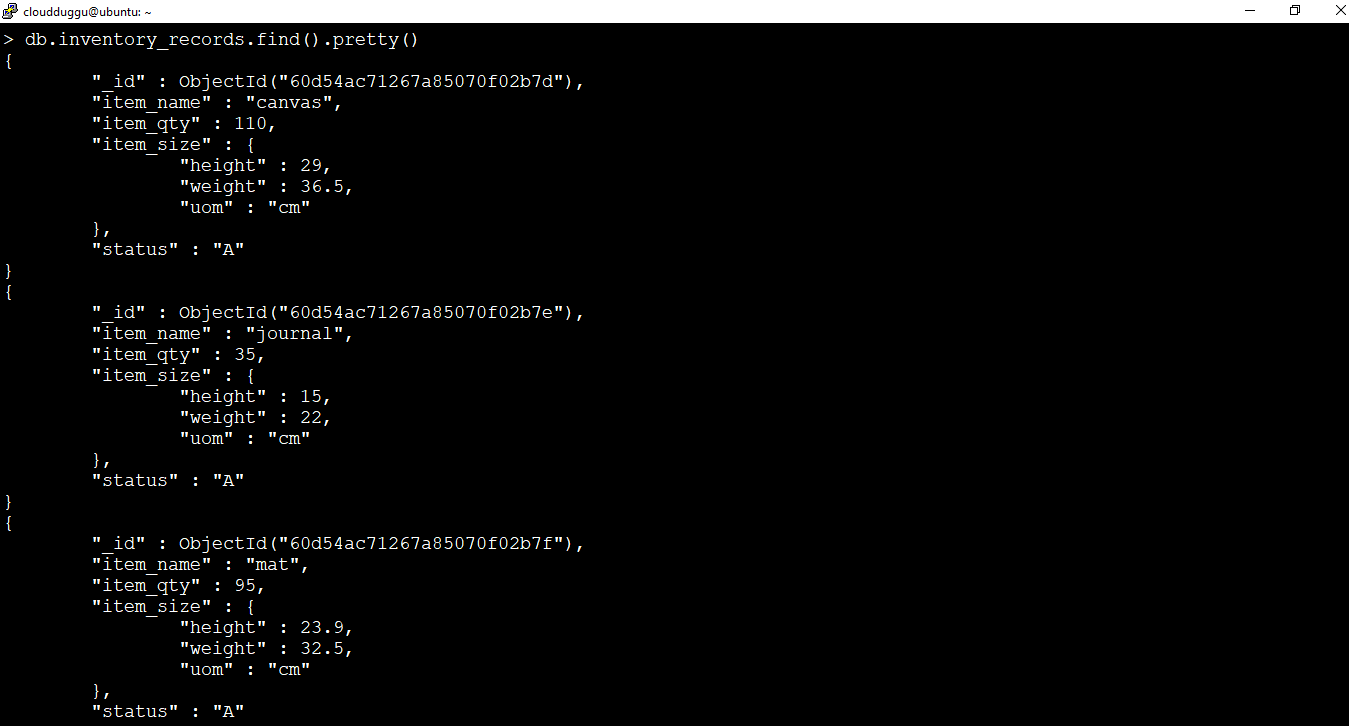
AND Condition in MongoDB
MongoDB supports the and condition to compare two Documents and return the result when both Documents conditions are matching. To use and condition we can use the $and operator.
Syntax:
The syntax of AND condition is as below.
Example:
Let us see the below example in which we are fetching all Documents where status equals "A" and item_qtyqty is less than 30.
In SQL language the same operation is written as below.
Output:
From the below output we can see only the below Document is qualifying the and condition and the result is returned.

OR Condition in MongoDB
In MongoDB or condition takes two Documents and check if either of the condition is matching then it returns the matching Documents. We can use the MongoDB < or condition using the $or operator.
Syntax:
The syntax of OR condition is as below.
Example:
Let us see the below example in which All Documents are returned where the status equals to "D" or item_qty is less than 30.
We can write the same operation in SQL as mentioned below.
Output:
We can see in the below output that all matching Documents are returned.

AND & OR Condition Together in MongoDB
Let us see the example of and & or condition together in which we are fetching all Documents where the status=p and item_qty are less than 80 or the item_name starts with the P character.
Example:
status: "P",
$or: [ { item_qty: { $lt: 80 } }, { item_name: /^p/ } ]
} )
In the SQL the operation is written as below.
Output:
The Matching Documents are returned in the below output.

NOT Condition in MongoDB
The MongoDB NOT condition applies the logical NOT operation and returns the Documents which are not matching.
Syntax:
The syntax of NOT condition is as below.
Example:
In the below example we will use the NOT operator to fetch the Documents where the item_qty is less than or equal to 60.
The SQL version of the same operation is as below.
Output:
