MongoDB provides the delete method to delete one Document or multiple Documents in a Collection. In this section, we will go through the MongoDB delete method.
The following delete method is supported by MongoDB.
- db.collection_name.deleteOne()
- db.collection_name.deleteMany()
Let us see each delete method in detail.
db.collection_name.deleteOne()
The MongoDB deleteOne() method is used to delete a single Document from the Collection.
Syntax:
The following is the syntax of deleteOne() method.
Let us see the example of deleteOne() method. We have a Collection name "item_inventory" present under the database "mongodb_test" that we created in the Insert Document section. We will use the "item_inventory" Collection to delete one Document where the item_name is equal to "journal".
Example :
Output:
We can see from the below output that the Document where item_name is qual to "journal" is deleted.
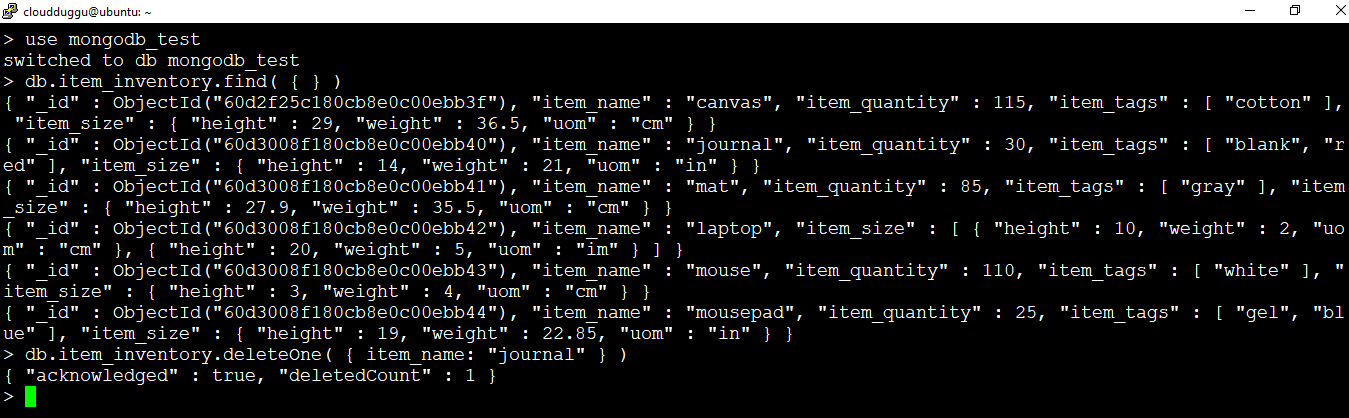
db.collection_name.deleteMany()
The MongoDB deleteMany() method is used to delete all Documents from a Collection if no filter condition is provided. If we pass a filter condition then it will delete all of the matching Documents.
Syntax:
The below is the syntax of deleteMany() method of MongoDB.
db.collection_name.deleteMany({}) /* This Syntax will delete all Docuemnts from the Collection. */
In the below example we will use the deleteMany method with filter and without filter condition to delete Documents. With filter condition, we will delete all Documents where item_name is equal to "mouse", and without filter condition, we will delete all Documents of the "item_inventory" Collection.
Example :
> db.item_inventory.deleteMany( { } )
Output:
