In this section of the MongoDB tutorial, we will go through the Insert() method.
In MongoDB, there is the following Insert() Method which is used to insert the documents in the collection.
- db.collection.insertOne()
- db.collection.insertMany()
- db.collection.insert()
Let us see each Insert() Method in detail.
db.collection.insertOne()
The insertOne() method is used to insert a single document in the collection. In case the collection does not exist then MongoDB Insert methods will create one.
Syntax:
The following is the syntax of insertOne() method.
db.collection.insertOne(
<document>,
{
writeConcern: <document>
}
)
Let us see an example to insert a document in the item_inventory collection in the mongodb_test database. We will use MongoDB Shell to perform this activity. Let's start the MongoDB Shell using mongo command as mentioned below and run the example.
Example:
cloudduggu@ubuntu:~$ mongo
> use mongodb_test
> db.item_inventory.insertOne(
{ item_name: "canvas", item_quantity: 115, item_tags: ["cotton"], item_size: { height: 29, weight: 36.5, uom: "cm" } }
)
Output:
We can see from the below snapshot that the Document has been inserted into the item_inventory collection which is present under the mongodb_test database.
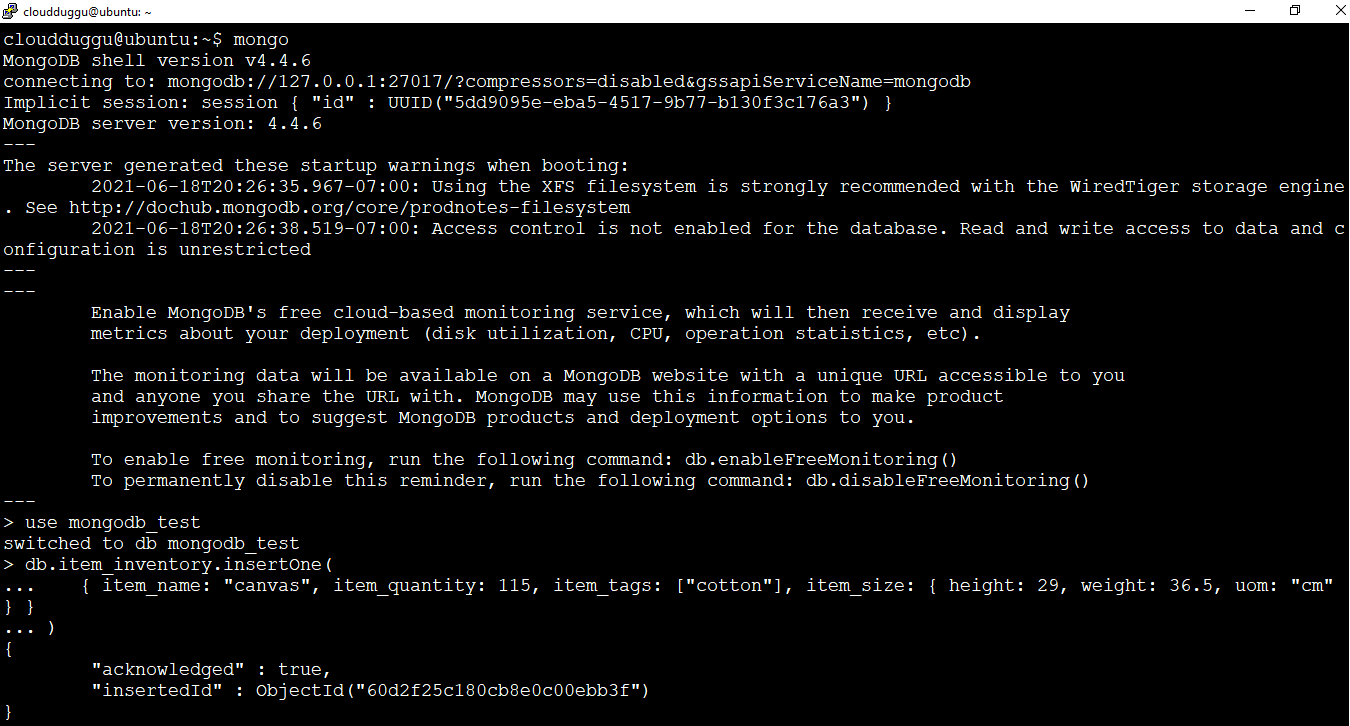
We will use the find() method to fetch the Document that we have inserted.
Example:
Output:
In the below output we can see that detail of the Document.

db.collection.insertMany()
The insertMany() method is used to insert many Documents in the Collection.
Syntax:
The insertMany() has the following syntax.
[
{
writeConcern: <document>,
ordered: <boolean>
}
)
Now, let's insert multiple documents into the item_inventory collection in the mongodb_test database using the below commands.
Example:
[ { item_name: "journal", item_quantity: 25, item_tags: ["blank", "red"], item_size: { height: 14, weight: 21, uom: "cm" } },
{ item_name: "mat", item_quantity: 85, item_tags: ["gray"], item_size: { height: 27.9, weight: 35.5, uom: "cm" } },
{ item_name: "laptop", item_quantity: 100, item_tags: ["black"], item_size: { height: 10, weight: 2, uom: "cm" } },
{ item_name: "mouse", item_quantity: 110, item_tags: ["white"], item_size: { height: 3, weight: 4, uom: "cm" } },
{ item_name: "mousepad", item_quantity: 25, item_tags: ["gel", "blue"], item_size: { height: 19, weight: 22.85, uom: "cm" } }
] )
Output:
We can see from the below output that multiple Documents are inserted in the item_inventory collection and each Document is assigned a unique ObjectId.
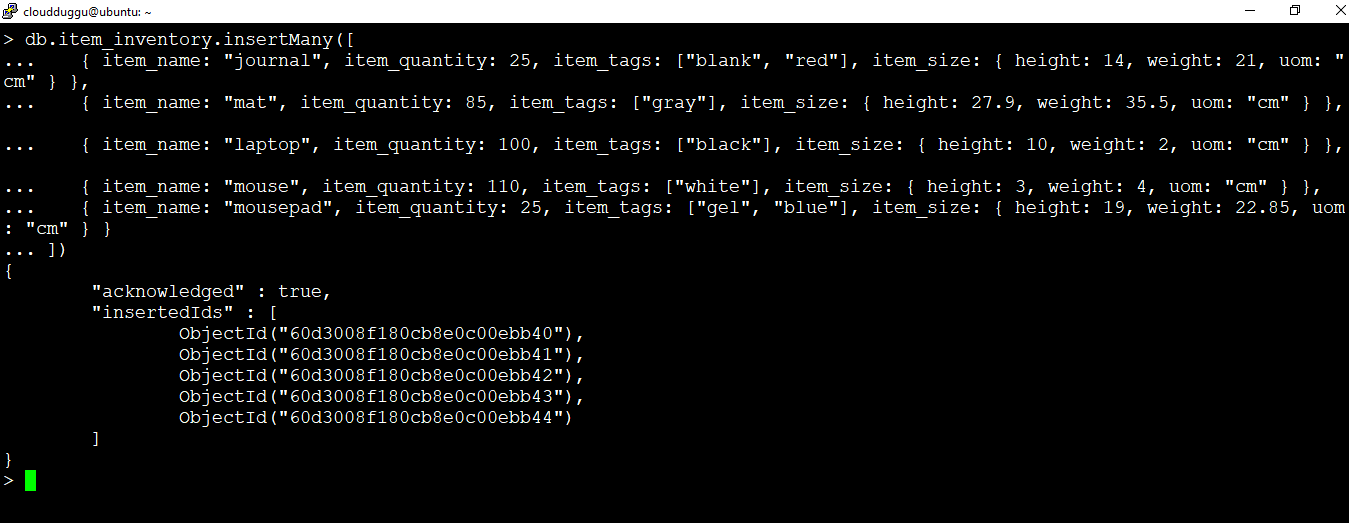
We will use the find( {} ) method to view the complete list of Documents in a Collection item_inventory.
Example:
Output:
We can see the complete list of Documents from the item_inventory Collection.
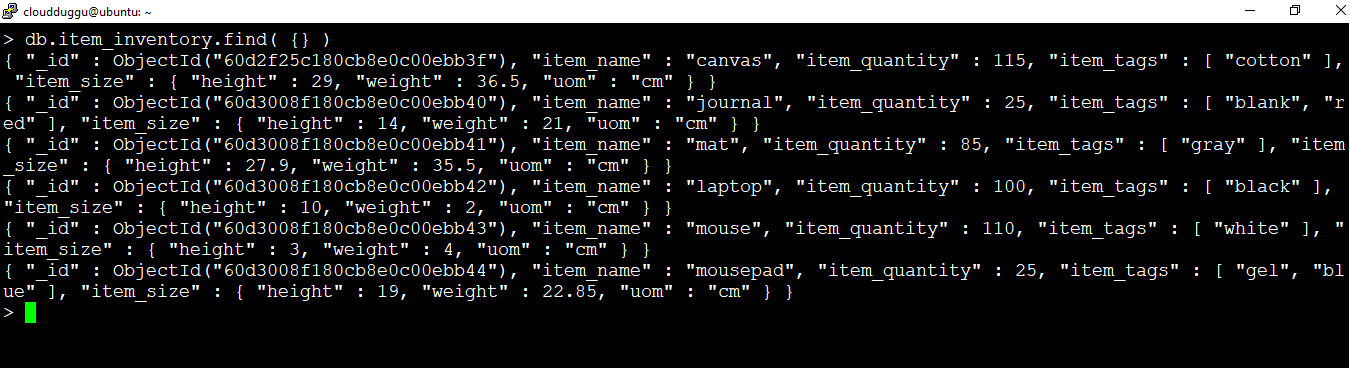
db.collection.insert()
The insert() method is used to insert a Document or multiple Documents in the Collection.
Syntax:
The insert() method has the following syntax.
<document or collection of documents>,
{
writeConcern: <document>,
ordered: <boolean>
}
)
Let us use the example of insert() method to insert a Document and multiple Documents in the Collection.
Insert a Single Document Using db.collection.insert()
Let us insert a single Document in the "products_detail" collection using the below command.
Example:
Output:
We can see that the single Document has been inserted into the Collection "products_detail".

Insert Multiple Document Using db.collection.insert()
We will insert multiple Documents in the "products_detail" collection using the below command.
Example:
[ { item_name: "pencil", item_quantity: 60, item_type: "no.2" },
{ item_name: "pen", item_quantity: 30 },
{ item_name: "eraser", item_quantity: 65 },
{ item_name: "notebook", item_quantity: 100 },
{ item_name: "pen", item_quantity: 110 }
] )
Output:
We can see the Documents are inserted in the Collection "products_detail".
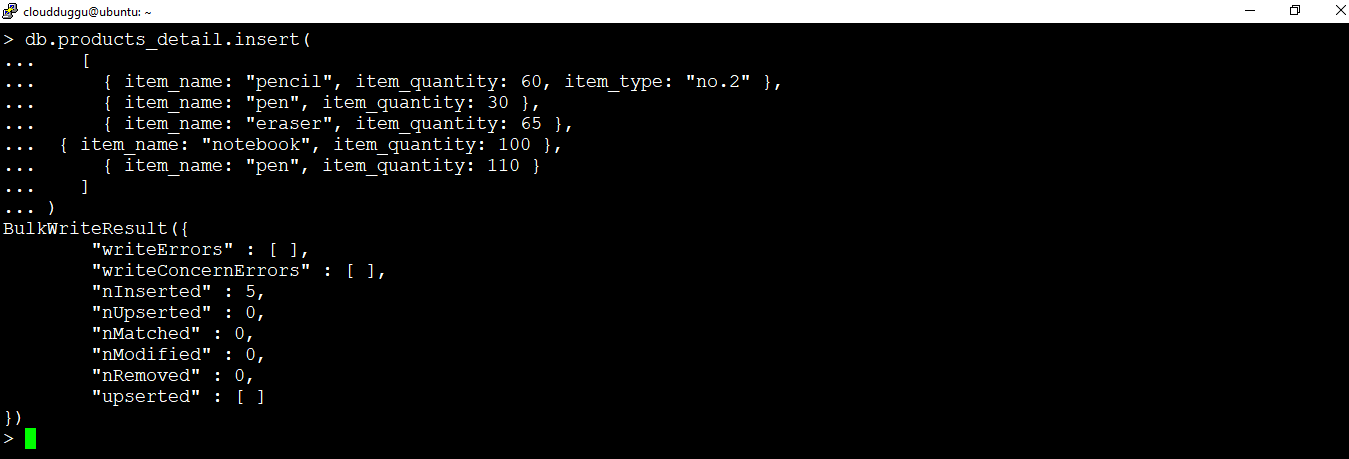