In this section of the tutorial, we will go through the Limit() and sort() methods of MongoDB.
- Limit() Method
- sort() Method
Let us see each method in detail in the below section. We have the following Collection name "inventory_records" that is created in the Select Document section and present in the "mongodb_test" database. We will use this Collection to perform the Limit and sort operation.
{ item_name: "canvas",item_qty: 110,item_size: { height: 29, weight: 36.5, uom: "cm" }, status: "A" },
{ item_name: "journal",item_qty: 35, item_size: { height: 15, weight: 22, uom: "cm" }, status: "A" },
{ item_name: "mat",item_qty: 95, item_size: { height: 23.9, weight: 32.5, uom: "cm" }, status: "A" },
{ item_name: "mousepad",item_qty: 45, item_size: { height: 16, weight: 28.85, uom: "cm" }, status: "P" },
{ item_name: "notebook",item_qty: 60, item_size: { height: 7.5, weight: 10, uom: "in" }, status: "P" },
{ item_name: "paper",item_qty: 200, item_size: { height: 9.5, weight: 12, uom: "in" }, status: "D" },
{ item_name: "planner",item_qty: 75, item_size: { height: 25.85, weight: 40, uom: "cm" }, status: "D" },
{ item_name: "postcard",item_qty: 25, item_size: { height: 15, weight: 17.25, uom: "cm" }, status: "A" },
{ item_name: "sketchbook",item_qty: 90, item_size: { height: 13, weight: 23, uom: "cm" }, status: "A" },
{ item_name: "sketch_pad",item_qty: 85, item_size: { height: 25.85, weight: 32.5, uom: "cm" }, status: "A" }
] );
Limit() Method
The MongoDB limit() method is used to limit the number of Documents that will be shown. It accepts a number that represents the number of Documents that will be projected.
Syntax:
The Following is the syntax of limit() method.
In the following example, we have used the find() method initially without parameter to see the complete Document of the "inventory_records" Collection, and then we have used the limit() method to show only 4 Documents.
Example:
> db.inventory_records.find().limit(4)
Output:
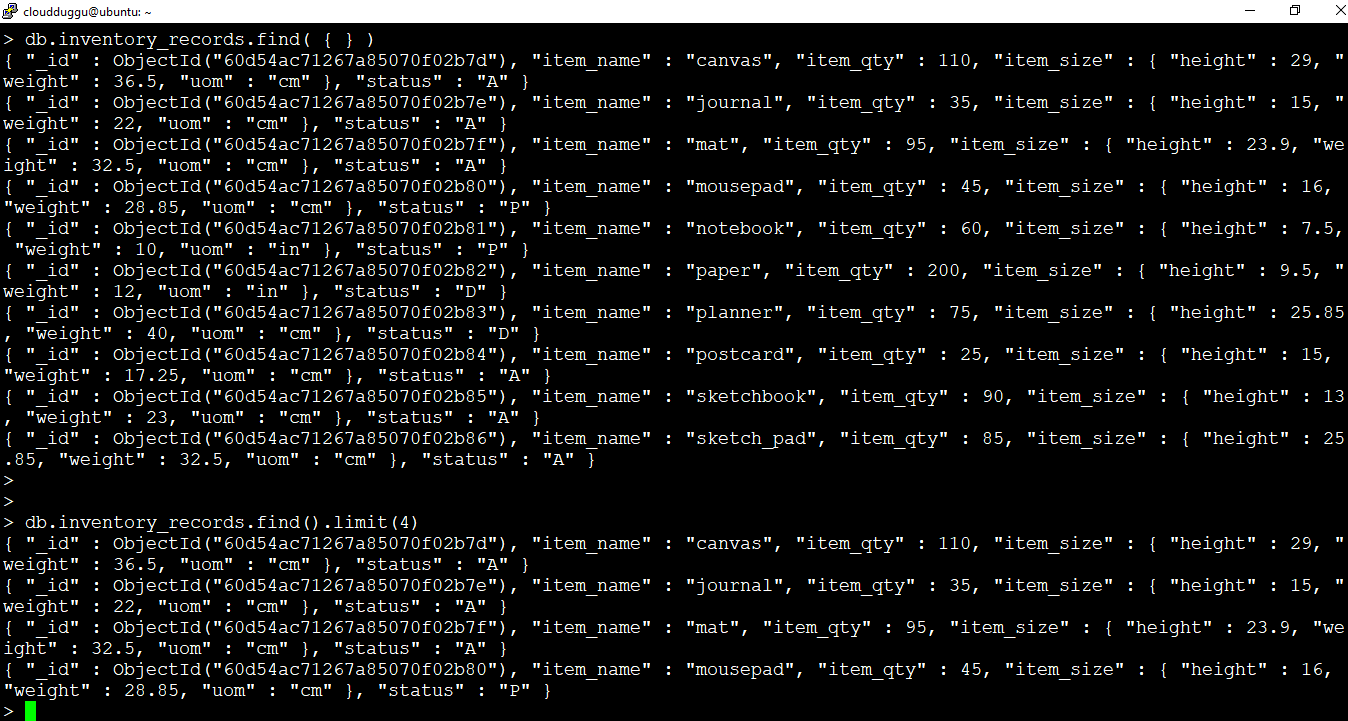
sort() Method
The MongoDB sort() method is used to perform the sorting of the input Document and return their sorted order. The sort() method provides two types of sort order, the first one is 1 that sort the Document in ascending order, and the second one is -1 that sort the Document in descending order.
Syntax:
The Following is the syntax of sort() method.
In the below example, we will use the sort() method to sort the "item_qty" Document of "inventory_records" Collection in ascending(1) and descending(-1) order.
Example:
> db.inventory_records.find().sort({"item_qty": -1})
Output:
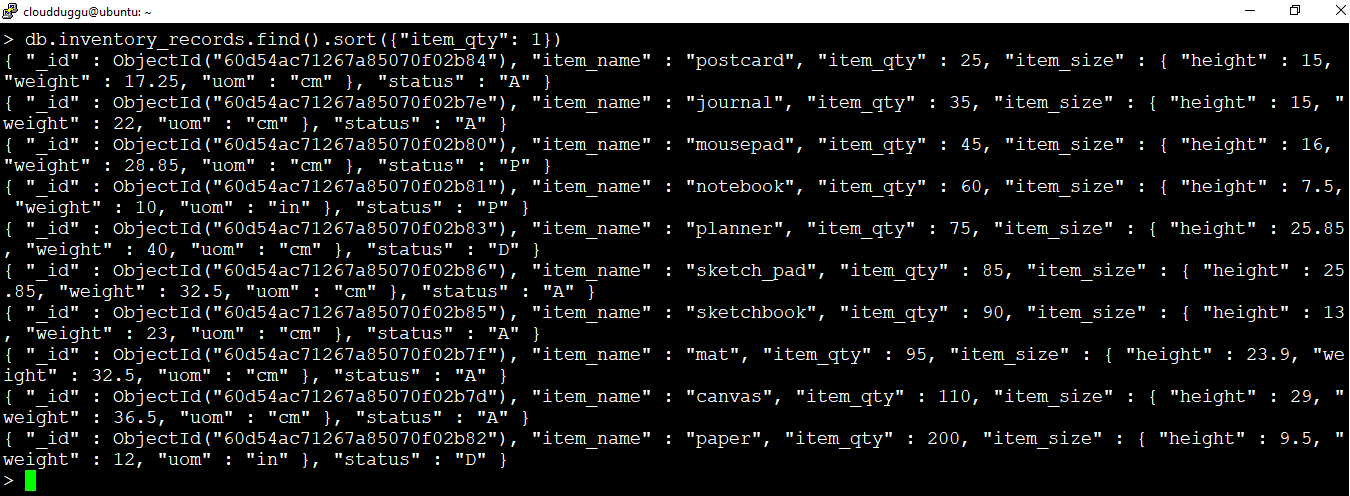
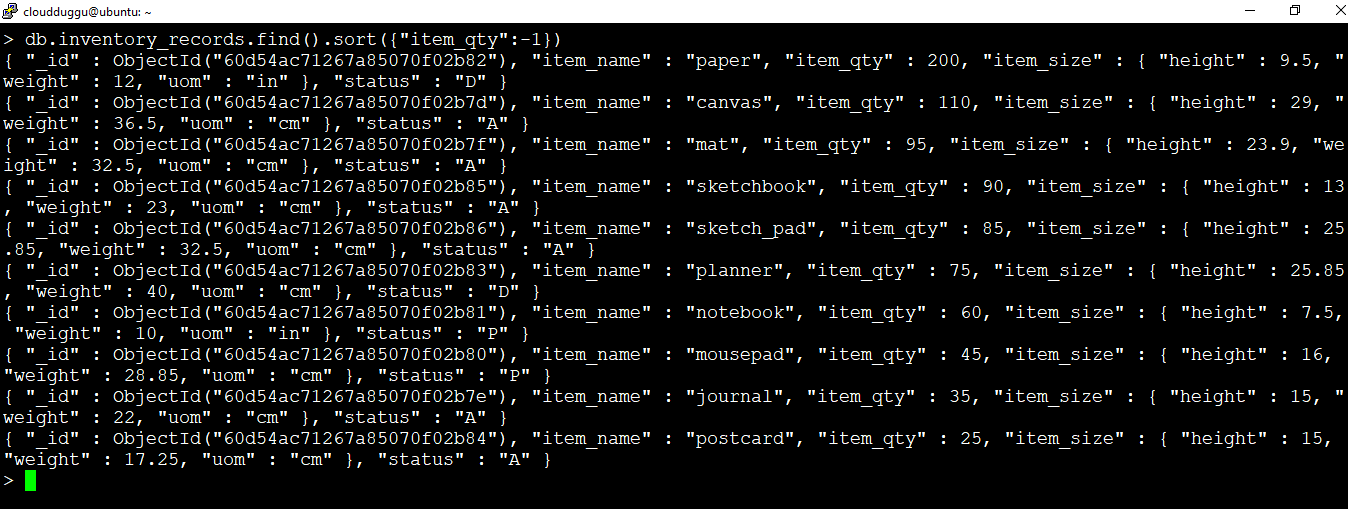